Dumper
Every debugging developer is a good friend with the function var_dump
, which lists all contents of any variable in
detail. Unfortunately, its output is without HTML formatting and outputs the dump into a single line of HTML code, not to mention
context escaping. It is necessary to replace the var_dump
with a more handy function. That is just what
dump()
is.
$arr = [10, 20.2, true, null, 'hello'];
dump($arr);
// or Debugger::dump($arr);
generates the output:
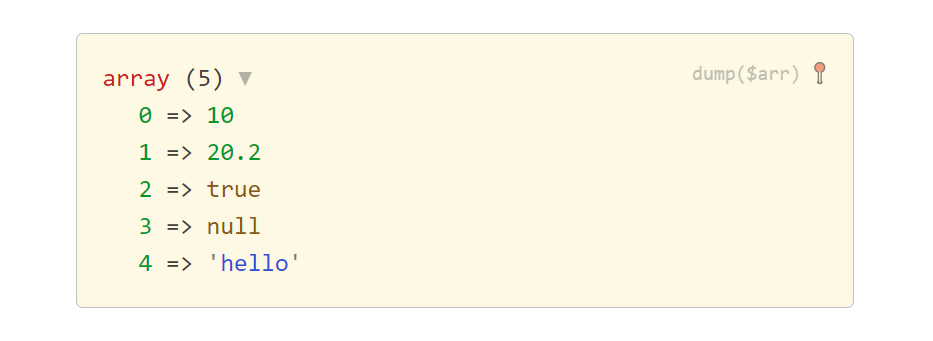
You can change the default light theme to dark:
Debugger::$dumpTheme = 'dark';
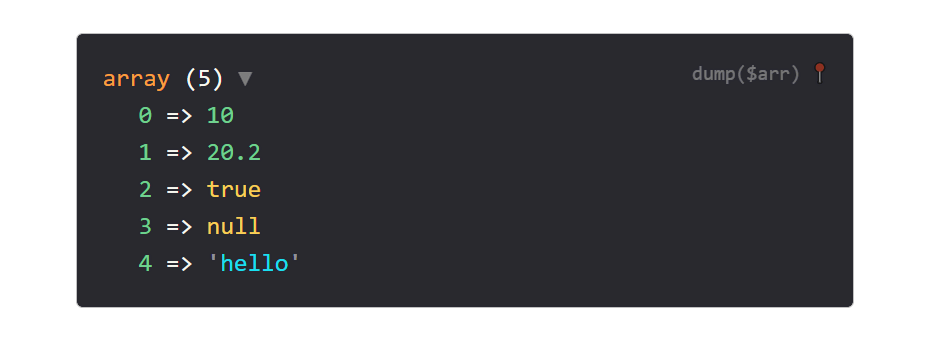
You can also change the nesting depth by Debugger::$maxDepth
and displayed strings length by
Debugger::$maxLength
. Naturally, lower values accelerate Tracy rendering.
Debugger::$maxDepth = 2; // default: 3
Debugger::$maxLength = 50; // default: 150
The dump()
function can display other useful information. Tracy\Dumper::LOCATION_SOURCE
adds a
tooltip with path to the file, where the function was called. Tracy\Dumper::LOCATION_LINK
adds a link to the file.
Tracy\Dumper::LOCATION_CLASS
adds a tooltip to every dumped object containing path to the file, in which the
object's class is defined. All these constants can be set in Debugger::$showLocation
variable before calling the
dump()
. You can set multiple values at once using the |
operator.
Debugger::$showLocation = Tracy\Dumper::LOCATION_SOURCE; // Shows path to where the dump() was called
Debugger::$showLocation = Tracy\Dumper::LOCATION_CLASS | Tracy\Dumper::LOCATION_LINK; // Shows both paths to the classes and link to where the dump() was called
Debugger::$showLocation = false; // Hides additional location information
Debugger::$showLocation = true; // Shows all additional location information
Very handy alternative to dump()
is dumpe()
(ie. dump and exit) and bdump()
. This allows
us to dump variables in Tracy Bar. This is useful, because dumps don't mess up the output and we can also add a title to
the dump.
bdump([2, 4, 6, 8], 'even numbers up to ten');
bdump([1, 3, 5, 7, 9], 'odd numbers up to ten');
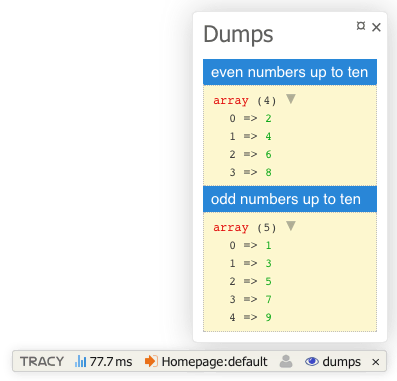